- A bitmap graphics context allows you to paint RGB colors, CMYK colors, or grayscale into a bitmap. A bitmap is a rectangular array (or raster) of pixels, each pixel representing a point in an image. Bitmap images are also called sampled images. See Creating a Bitmap Graphics Context.
- A PDF graphics context allows you to create a PDF file. In a PDF file, your drawing is preserved as a sequence of commands. There are some significant differences between PDF files and bitmaps:
- PDF files, unlike bitmaps, may contain more than one page.
- When you draw a page from a PDF file on a different device, the resulting image is optimized for the display characteristics of that device.
- PDF files are resolution independent by nature—the size at which they are drawn can be increased or decreased infinitely without sacrificing image detail. The user-perceived quality of a bitmap image is tied to the resolution at which the bitmap is intended to be viewed.
- A window graphics context is a graphics context that you can use to draw into a window. Note that because Quartz 2D is a graphics engine and not a window management system, you use one of the application frameworks to obtain a graphics context for a window. See Creating a Window Graphics Context in Mac OS X for details.
- A layer context (
CGLayerRef
) is an offscreen drawing destination associated with another graphics context. It is designed for optimal performance when drawing the layer to the graphics context that created it. A layer context can be a much better choice for offscreen drawing than a bitmap graphics context. See Core Graphics Layer Drawing. - When you want to print in Mac OS X, you send your content to a PostScript graphics context that is managed by the printing framework. SeeObtaining a Graphics Context for Printing for more information.
The opaque data types available in Quartz 2D include the following:
CGImageRef
, used to represent bitmap images and bitmap image masks based on sample data that you supply. See Bitmap Images and Image Masks.CGLayerRef
, used to represent a drawing layer that can be used for repeated drawing (such as for backgrounds or patterns) and for offscreen drawing. See Core Graphics Layer DrawingCGPatternRef
, used for repeated drawing. See Patterns.CGFunctionRef
, used to define callback functions that take an arbitrary number of floating-point arguments. You use this data type when you create gradients for a shading. See Gradients.CGColorRef
andCGColorSpaceRef
, used to inform Quartz how to interpret color. See Color and Color Spaces.CGImageSourceRef
andCGImageDestinationRef
, which you use to move data into and out of Quartz. See Data Management in Quartz 2D andImage I/O Programming Guide.CGPDFDictionaryRef
,CGPDFObjectRef
,CGPDFPageRef
,CGPDFStream
,CGPDFStringRef
, andCGPDFArrayRef
, which provide access to PDF metadata. See PDF Document Creation, Viewing, and Transforming.CGPSConverterRef
, used to convert PostScript to PDF. It is not available in iOS. See PostScript Conversion.
Graphics States
The graphics context contains a stack of graphics states. When Quartz creates a graphics context, the stack is empty. When you save the graphics state, Quartz pushes a copy of the current graphics state onto the stack. When you restore the graphics state, Quartz pops the graphics state off the top of the stack. The popped state becomes the current graphics state.
To save the current graphics state, use the function
CGContextSaveGState
to push a copy of the current graphics state onto the stack. To restore a previously saved graphics state, use the function CGContextRestoreGState
to replace the current graphics state with the graphics state that’s on top of the stack.
Quartz 2D Coordinate Systems
- In iOS, a drawing context returned by an
UIView
. - In iOS, a drawing context created by calling the
UIGraphicsBeginImageContextWithOptions
function.
Rotation
To draw a box rotated by 45 degrees, you rotate the coordinate system of the page (the CTM) before you draw the box. Quartz draws to the output device using the rotated coordinate system.
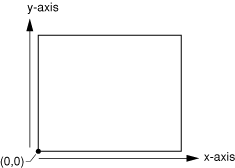
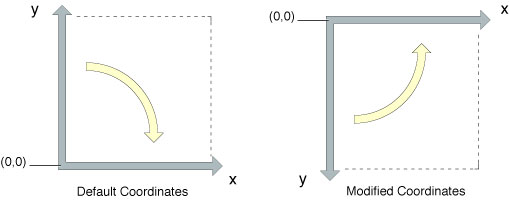
-1
.UIImage
object to wrap a CGImage
object you create, you do not need to modify the CTM. The UIImage
object automatically compensates for the modified coordinate system applied by UIKit.Modifying the Current Transformation Matrix
CGContextDrawImage (myContext, rect, myImage); |
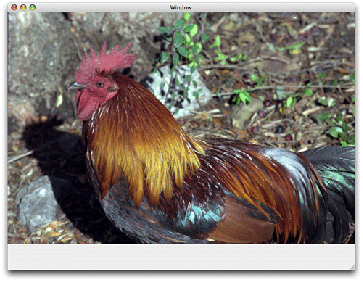
Translation moves the origin of the coordinate space by the amount you specify for the x and y axes. You call the function
CGContextTranslateCTM
to modify the x and y coordinates of each point by a specified amount. Figure 5-3 shows an image translated by 100 units in the x-axis and 50 units in the y-axis, using the following line of code:CGContextTranslateCTM (myContext, 100, 50); |
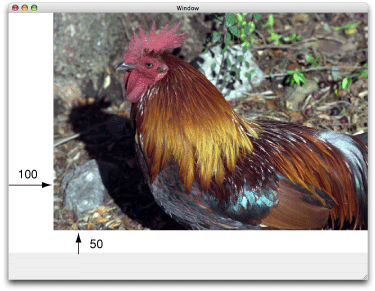
Rotation moves the coordinate space by the angle you specify. You call the function
CGContextRotateCTM
to specify the rotation angle, in radians.Figure 5-4 shows an image rotated by –45 degrees about the origin, which is the lower left of the window, using the following line of code:CGContextRotateCTM (myContext, radians(–45.)); |
The image is clipped because the rotation moved part of the image to a location outside the context. You need to specify the rotation angle in radians.
It’s useful to write a radians routine if you plan to perform many rotations.
#include <math.h> |
static inline double radians (double degrees) {return degrees * M_PI/180;} |
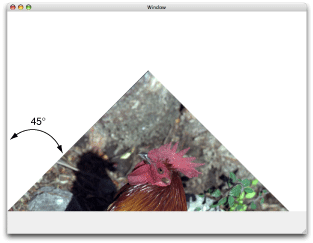
Scaling changes the scale of the coordinate space by the x and y factors you specify, effectively stretching or shrinking the image. The magnitude of the x and y factors governs whether the new coordinates are larger or smaller than the original. In addition, by making the x factor negative, you can flip the coordinates along the x-axis; similarly, you can flip coordinates horizontally, along the y-axis, by making the y factor negative. You call the function
CGContextScaleCTM
to specify the x and y scaling factors. Figure 5-5 shows an image whose x values are scaled by .5 and whose y values are scaled by .75, using the following line of code:CGContextScaleCTM (myContext, .5, .75); |
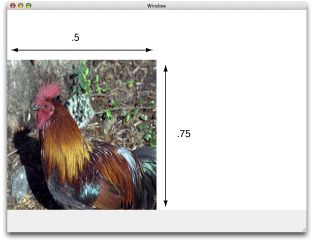
Concatenation combines two matrices by multiplying them together. You can concatenate several matrices to form a single matrix that contains the cumulative effects of the matrices. You call the function
CGContextConcatCTM
to combine the CTM with an affine transform. Affine transforms, and the functions that create them, are discussed in Creating Affine Transforms.
Another way to achieve a cumulative effect is to perform two or more transformations without restoring the graphics state between transformation calls.Figure 5-6 shows an image that results from translating an image and then rotating it, using the following lines of code:
CGContextTranslateCTM (myContext, w,h); |
CGContextRotateCTM (myContext, radians(-180.)); |
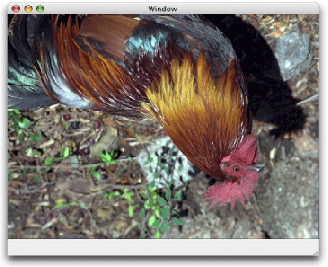
Rotation Affine Transform
CGAffineTransform centerTransform = CGAffineTransformMakeTranslation(scaleSize.width/2, scaleSize.height/2);
CGAffineTransform rotateTransform = CGAffineTransformRotate(centerTransform, -(rotation * M_PI / 180));
CGAffineTransform recenterTransform = CGAffineTransformTranslate(rotateTransform, -scaleSize.width/2, -scaleSize.height/2);
CGAffineTransform finalTransform = CGAffineTransformScale(recenterTransform,
scaleSize.width/originalPdfRect.size.width,
scaleSize.height/originalPdfRect.size.height);
http://stackoverflow.com/questions/15696850/cgcontext-rotate-rectangle
http://stackoverflow.com/questions/15696850/cgcontext-rotate-rectangle